Introduction
React JS is one of the most in-demand JavaScript libraries for front-end development, making it a crucial skill for developers looking to land high-paying jobs. Whether you’re a fresher or an experienced professional, preparing for a React JS interview requires a solid grasp of fundamental and advanced concepts. In this guide, we’ll cover frequently asked interview questions, coding challenges, best practices, and portfolio-building strategies to help you ace your interview.
Apply now and take your first step towards a successful career in Web Development! Placement Guarantee Program
Understanding React JS Concepts
A strong foundation in React JS concepts is essential for clearing interviews confidently. Here are some key areas you need to focus on:
- Virtual DOM: React’s Virtual DOM enhances performance by updating only the necessary parts of the real DOM.
- JSX: JavaScript XML, or JSX, allows you to write HTML-like syntax within JavaScript.
- State and Props: Understanding the difference between state (component-level data) and props (data passed from parent to child components) is crucial.
- React Hooks: Hooks like
useState
,useEffect
, anduseContext
help manage state and side effects in functional components. - Component Lifecycle: Knowing lifecycle methods like
componentDidMount
andcomponentDidUpdate
is essential for working with class components.
Apply now and take your first step towards a successful career in Web Development! Placement Guarantee Program
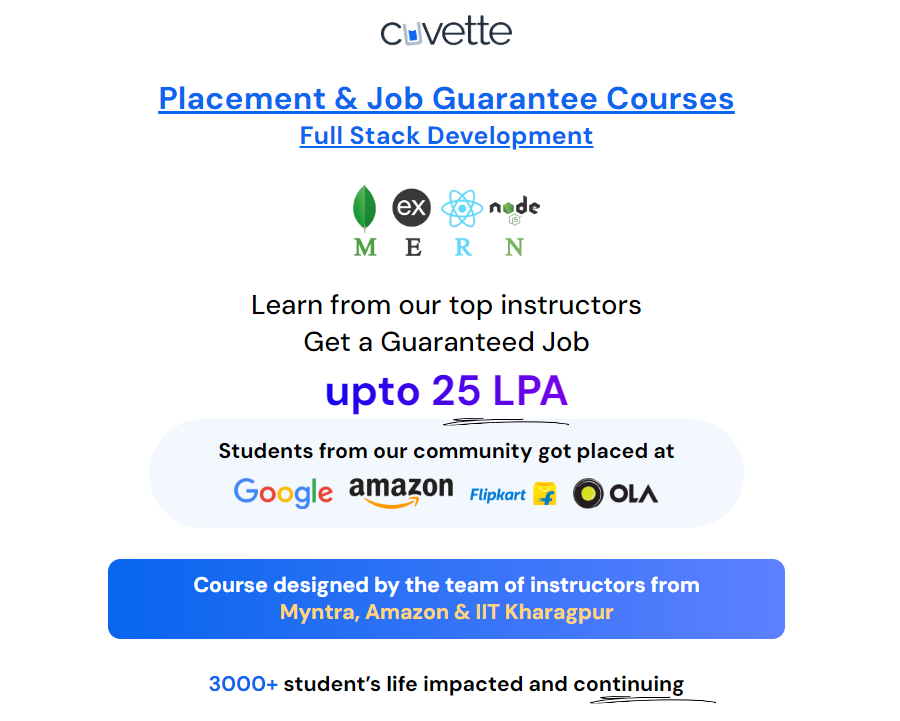
Identifying Key Areas of Focus
React JS interviews often focus on the following topics:
- JSX and Rendering: How does JSX work in React?
- React Router: How do you handle routing in a React application?
- State Management: Comparison of Redux, Context API, and Recoil.
- Styling Components: Different approaches such as CSS-in-JS, Styled Components, and Tailwind CSS.
- Performance Optimization: Techniques like code splitting, lazy loading, and memoization to improve performance.
Practicing Coding Challenges
To excel in technical rounds, practice coding challenges such as:
- Implement a Counter with Hooks
- Fetch and Display API Data
- Build a Simple To-Do App with React
- Debounce Input Handling
- Optimize a React Application for Performance
Hands-on practice is key to cracking React interviews.
Apply now and take your first step towards a successful career in Web Development! Placement Guarantee Program
Reviewing Best Practices and Advanced Techniques
To stand out in interviews, showcase knowledge of advanced concepts and best practices, such as:
- Code Splitting and Lazy Loading: Improve performance by loading components only when needed.
- Error Boundaries: Handle errors gracefully in React applications.
- Optimizing Rendering: Use
React.memo
,useCallback
, anduseMemo
to optimize performance. - Testing in React: Learn Jest and React Testing Library for writing unit tests.
- Security Best Practices: Prevent common vulnerabilities like XSS and CSRF attacks.
Building a Portfolio
A strong portfolio helps demonstrate your React JS expertise to potential employers. Include:
- A fully responsive personal website
- Real-world projects showcasing React skills
- Open-source contributions (e.g., GitHub projects)
- A blog or documentation about React concepts
Employers appreciate practical skills, so highlight your best projects!
Understanding the Interview Process
React JS interviews generally follow this structure:
- Technical Screening – Basic JavaScript and React concepts.
- Coding Round – Solve real-world coding problems.
- System Design Discussion – Discuss the architecture of a React application.
- Behavioral Interview – Showcase problem-solving and teamwork skills.
Comparing React with Other Technologies
React JS vs. Other Frameworks:
- React vs. Angular: React is component-based and flexible, while Angular is a full-fledged MVC framework.
- React vs. Vue: Vue is simpler for beginners, while React has a larger ecosystem.
- React vs. Svelte: Svelte compiles code at build time, whereas React relies on runtime updates.
Learning About Tools and Libraries
Enhance your React development skills by learning these tools:
- State Management: Redux, Zustand, Recoil.
- UI Libraries: Material-UI, Ant Design, Chakra UI.
- Testing Tools: Jest, React Testing Library, Cypress.
- Development Tools: ESLint, Prettier, Webpack, Vite.
Enhancing Problem-Solving Skills
To succeed in interviews, practice solving React-related problems, such as:
- Handling asynchronous operations efficiently.
- Debugging common errors in React components.
- Optimizing performance with efficient re-renders.
Apply now and take your first step towards a successful career in Web Development! Placement Guarantee Program
Staying Updated with Industry Trends
React is continuously evolving. Stay ahead by:
- Following React’s official blog and GitHub.
- Participating in React meetups and conferences.
- Keeping up with trends like server-side rendering (SSR) with Next.js.
- Learning about React Native for mobile development.
Beginner-Level React JS Interview Questions
- What is React JS?
- What are the advantages of using React?
- What is JSX, and why is it used in React?
- What is the difference between React and React Native?
- What are React components?
- What are functional components and class components?
- What is the difference between state and props?
- How do you create a functional component in React?
- What is the purpose of the
useState
hook? - How does React handle events?
- What is the virtual DOM?
- What are React fragments?
- What is the importance of keys in React lists?
- What is conditional rendering in React?
- How do you pass props between components?
- What is the purpose of the
useEffect
hook? - What is the difference between controlled and uncontrolled components?
- What is the default behavior of forms in React?
- What are synthetic events in React?
- How can you style components in React?
Intermediate-Level React JS Interview Questions
- What is the difference between
useState
anduseReducer
? - What are higher-order components (HOC)?
- What is the Context API in React?
- How does the
useContext
hook work? - What is prop drilling, and how do you avoid it?
- What are custom hooks in React?
- How do you use the
useRef
hook in React? - How does the
useMemo
hook help with performance optimization? - What is the difference between
useCallback
anduseMemo
? - How do you handle API calls in React?
- What is lazy loading in React?
- What are error boundaries in React?
- How do you optimize React applications for better performance?
- What is React Router, and how does it work?
- What is the difference between client-side and server-side rendering?
- How do you redirect a user in React Router?
- What is Redux, and how does it work?
- What are the core principles of Redux?
- What is the difference between Redux and Context API?
- How do you manage state using Redux?
Advanced-Level React JS Interview Questions
- How do you implement authentication in a React app?
- What is React Fiber, and how does it work?
- What are concurrent features in React 18?
- What is suspense in React?
- How does server-side rendering (SSR) improve performance?
- What is Next.js, and how does it enhance React applications?
- What are the best practices for structuring a large React application?
- What are Recoil and Zustand, and how do they compare to Redux?
- How do you implement internationalization (i18n) in a React app?
- How does React handle memory management?
- How do you use WebSockets in React?
- What is the role of React portals?
- What is hydration in React, and why is it important?
- How does React handle accessibility (a11y)?
- What are the benefits of using TypeScript with React?
- How do you implement dark mode in a React application?
- How does React compare with Angular and Vue.js?
- How do you test React applications using Jest and React Testing Library?
- What are shallow rendering and deep rendering in testing?
- How do you manage side effects in a React application?
React Hooks-Specific Questions
- Why were React Hooks introduced?
- What are the rules of Hooks?
- How does
useEffect
differ from lifecycle methods in class components? - What are dependency arrays in
useEffect
? - How do you use
useReducer
for complex state management? - How can you fetch data using
useEffect
? - What is the use of
useLayoutEffect
in React? - How does
useImperativeHandle
work? - Can you use multiple
useEffect
hooks in a component? - What is the difference between
useState
anduseRef
?
React Performance Optimization Questions
- How do you optimize re-renders in React?
- What are memoized components in React?
- What is
React.memo
, and how does it work? - What is the role of
shouldComponentUpdate
in class components? - How can you optimize a React application’s bundle size?
- What is tree shaking, and how does it work in React?
- How does React handle reconciliation?
- What is the significance of
key
props in lists? - How do you use code splitting in React?
- How do you prevent memory leaks in React components?
React Router-Specific Questions
- How do you configure dynamic routes in React Router?
- What is the difference between
useHistory
anduseNavigate
? - How do you pass parameters in React Router?
- How do you protect routes using authentication?
- How does nested routing work in React Router?
State Management-Specific Questions
- What are the core principles of Redux?
- How does Redux middleware like
redux-thunk
work? - How does Redux Toolkit simplify state management?
- What is the difference between Redux and MobX?
- How do you persist state in Redux?
Miscellaneous React Questions
- What is a design pattern in React?
- How do you handle browser storage (localStorage/sessionStorage) in React?
- How do you handle file uploads in React?
- What is the difference between SSR, CSR, and SSG?
- How do you implement infinite scrolling in React?
- How do you handle forms efficiently in React?
- How does React compare with Svelte?
- What are progressive web apps (PWAs), and how does React support them?
- How do you integrate third-party libraries in React?
- What is the future of React JS?
Preparing for a React JS interview requires a mix of theoretical knowledge, hands-on coding practice, and a well-structured portfolio. By focusing on key concepts, practicing common interview questions, and staying updated with industry trends, you can increase your chances of success.
If you’re looking for a structured way to learn React and get placed in top tech companies, join our Placement Guarantee Program today!
Apply now and take your first step towards a successful career in Web Development! Placement Guarantee Program
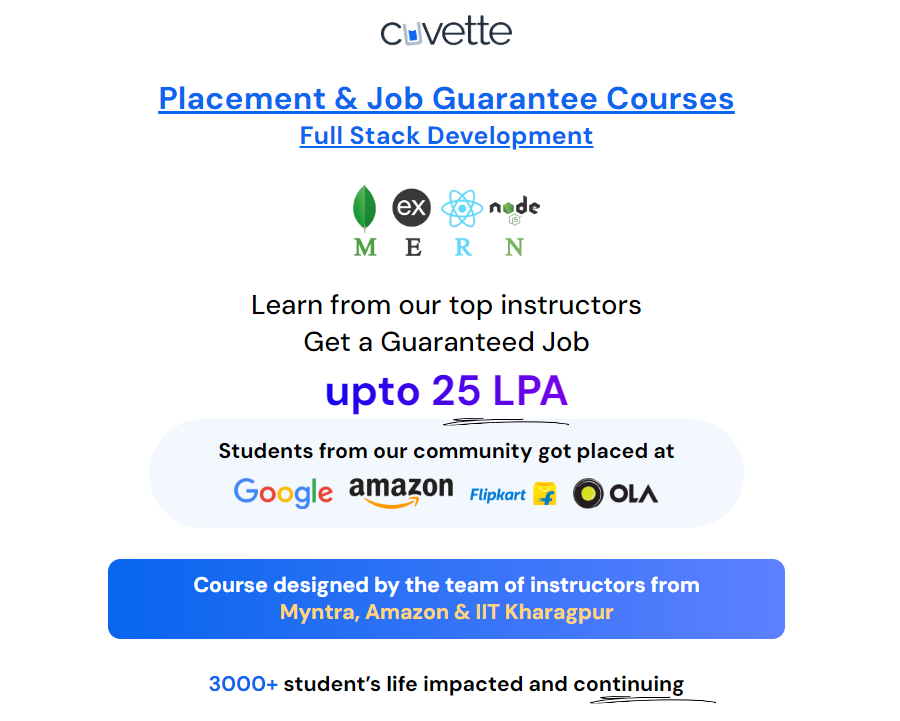
Recent Comments