The __init__
method in Python is a fundamental part of object-oriented programming. It acts as a constructor, allowing developers to initialize object attributes when an instance of a class is created. Understanding its purpose is crucial for writing efficient and maintainable Python code.
If you are also looking for jobs or taking the first step in your web development career, join our Placement Guaranteed Course designed by top IITians and Senior developers & get a Job guarantee of CTC up to 25 LPA – https://cuvette.tech/placement-guarantee-program
Why __init__
is Required in Python
The __init__
method ensures that every object starts with a predefined state. Without it, we would have to manually assign attributes every time we create an instance. It simplifies the process and makes code more readable.
The Role of __init__
in Object Creation
When a class is instantiated, Python automatically calls the __init__
method. This allows developers to set object attributes right at the moment of creation. For example:
class Student:
def __init__(self, name, age):
self.name = name
self.age = age
student1 = Student("Alice", 21)
print(student1.name) # Output: Alice
This example highlights how __init__
initializes attributes without additional lines of code.
Default and Parameterised Constructors
Python supports both default and parameterized constructors:
- Default Constructor: Does not accept parameters except
self
. - Parameterized Constructor: Accepts parameters to initialize attributes.
class Course:
def __init__(self, course_name="Python Basics"):
self.course_name = course_name
course1 = Course()
print(course1.course_name) # Output: Python Basics
This makes __init__
versatile for different use cases.

Best Practices for Using __init__
To write efficient Python classes, follow these best practices:
- Keep
__init__
concise—only initialize essential attributes. - Use default values to enhance flexibility.
- Avoid complex logic inside
__init__
; keep initialization separate from computation. - Ensure proper error handling when dealing with user inputs.
If you are also looking for jobs or taking the first step in your web development career, join our Placement Guaranteed Course designed by top IITians and Senior developers & get a Job guarantee of CTC up to 25 LPA – https://cuvette.tech/placement-guarantee-program
Crafting Concise __init__
Methods
A well-structured __init__
method improves code readability and efficiency. Instead of overloading it with logic, keep it clean and direct. For instance:
class Employee:
def __init__(self, name, salary=50000):
self.name = name
self.salary = salary
This makes the code easy to understand and maintain.
Initializing Objects with Specific Attributes
Setting attributes during object creation is essential for defining object behavior. Consider this example:
class DataScienceCourse:
def __init__(self, name, duration, fee):
self.name = name
self.duration = duration
self.fee = fee
course = DataScienceCourse("Machine Learning", "6 months", 30000)
print(course.name) # Output: Machine Learning
This showcases how __init__
helps in efficient object creation.
Examples through Real-World Scenarios
A practical example of using __init__
is in web development:
class User:
def __init__(self, username, email):
self.username = username
self.email = email
user1 = User("john_doe", "[email protected]")
Here, __init__
ensures each user has a unique profile upon creation.
Advanced Usage and Modular Code
For advanced applications, __init__
can be used alongside inheritance:
class Developer:
def __init__(self, name, language):
self.name = name
self.language = language
class BackendDeveloper(Developer):
def __init__(self, name, language, framework):
super().__init__(name, language)
self.framework = framework
This approach makes code more modular and reusable.
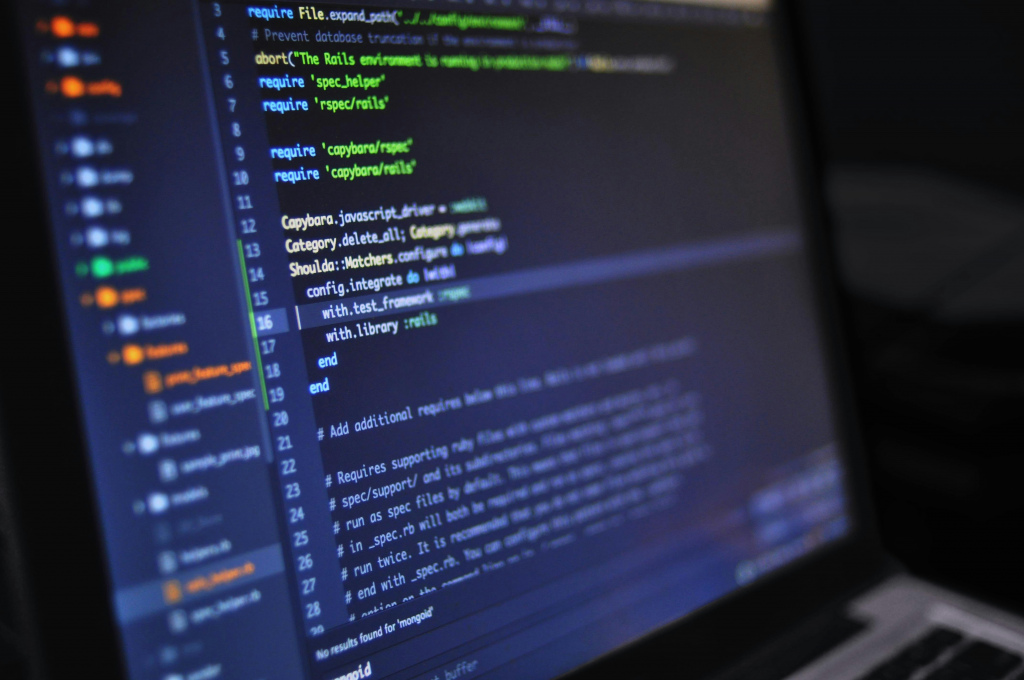
Troubleshooting Common Issues
Common errors with __init__
include:
- Forgetting
self
: All instance variables must useself
. - Incorrect parameter passing: Ensure the arguments match.
- Overloading complexity: Keep
__init__
straightforward.
If you are also looking for jobs or taking the first step in your web development career, join our Placement Guaranteed Course designed by top IITians and Senior developers & get a Job guarantee of CTC up to 25 LPA – https://cuvette.tech/placement-guarantee-program
FAQs
What is __init__()
in Python?
It is a special method that initializes a class object upon creation.
What is self
in Python?
self
represents the instance of the class and allows access to its attributes and methods.
Why is __init__
required in Python?
It ensures that each instance starts with a predefined state, improving efficiency and structure.
What is the difference between default and parameterized constructors?
A default constructor has no parameters, while a parameterized constructor allows initialization with specific values.
Conclusion
Understanding __init__
is essential for Python development. It simplifies object initialization, enhances code organization, and improves efficiency. By following best practices, you can write cleaner and more maintainable Python code. Start implementing __init__
effectively in your projects today.
If you are also looking for jobs or taking the first step in your web development career, join our Placement Guaranteed Course designed by top IITians and Senior developers & get a Job guarantee of CTC up to 25 LPA – https://cuvette.tech/placement-guarantee-program
Recent Comments