Understanding Set Basics
Sets in Python are a powerful data structure that store unique elements in an unordered manner. Unlike lists and tuples, sets do not allow duplicate values, making them ideal for scenarios that require distinct elements. Additionally, they offer efficient operations for mathematical set theory, such as union, intersection, and difference.
Python sets are mutable, meaning you can modify their contents by adding or removing elements. However, individual elements within a set must be immutable data types like numbers, strings, or tuples. Understanding sets can enhance your ability to handle and process data efficiently.
What Are Sets?
Sets are defined as an unordered collection of unique elements. Since they do not maintain a specific order, indexing and slicing are not possible. Sets are commonly used in scenarios where duplicates must be eliminated or where mathematical set operations are required.
Key Characteristics of Sets:
- Unordered: Elements have no fixed order.
- Unique Elements: Duplicate values are automatically removed.
- Mutable: You can add or remove elements after creation.
- Efficient Operations: Provides fast membership testing and mathematical set operations.
Creating Sets
Python provides multiple ways to create sets. The two primary methods include using curly braces {}
and the set()
function.
Using Curly Braces
my_set = {1, 2, 3, 4}
print(my_set) # Output: {1, 2, 3, 4}
Using the set()
Function
my_set = set([1, 2, 3, 4, 4, 5])
print(my_set) # Output: {1, 2, 3, 4, 5}
The set()
function is particularly useful when converting other iterables, such as lists or strings, into sets.
Set Operations
Python sets support various mathematical operations to perform comparisons and modifications efficiently.
Union of Sets
The union operation combines two sets and returns a new set containing all unique elements.
set1 = {1, 2, 3}
set2 = {3, 4, 5}
result = set1.union(set2)
print(result) # Output: {1, 2, 3, 4, 5}
Intersection of Sets
The intersection operation returns only the common elements between two sets.
set1 = {1, 2, 3}
set2 = {3, 4, 5}
result = set1.intersection(set2)
print(result) # Output: {3}
Modifying Sets
You can modify sets using various methods like add()
, remove()
, and clear()
.
Adding and Removing Elements
my_set = {1, 2, 3}
my_set.add(4)
print(my_set) # Output: {1, 2, 3, 4}
my_set.remove(2)
print(my_set) # Output: {1, 3, 4}
Iterating Through Sets
Since sets are unordered, looping through them ensures access to all elements without a specific order.
my_set = {1, 2, 3, 4}
for item in my_set:
print(item)
Handling Duplicate Values
Sets automatically eliminate duplicate values. If duplicate elements are added to a set, they are ignored.
my_set = {1, 2, 2, 3, 4}
print(my_set) # Output: {1, 2, 3, 4}
Set Methods and Operators
Python offers various methods and operators for working with sets efficiently.
Method | Description |
---|---|
union() | Returns a new set containing all unique elements |
intersection() | Returns common elements between sets |
difference() | Returns elements present in one set but not the other |
symmetric_difference() | Returns elements that are in either set, but not both |
Order and Sorting
Unlike lists, sets are unordered collections. However, you can sort a set using sorted()
, which returns a list of sorted elements.
my_set = {3, 1, 4, 2}
print(sorted(my_set)) # Output: [1, 2, 3, 4]
Practical Examples
Sets are widely used in real-world applications such as:
- Removing Duplicates: Converting a list to a set removes duplicates effortlessly.
- Fast Membership Testing: Checking if an element exists in a set is significantly faster than in a list.
- Mathematical Operations: Useful for data comparisons, filtering, and merging datasets.
Troubleshooting Common Issues
Key Errors
Attempting to remove an element that does not exist raises a KeyError
.
my_set = {1, 2, 3}
my_set.remove(4) # Raises KeyError
To avoid this, use discard()
instead.
my_set.discard(4) # No error, even if 4 is not in the set
FAQs
What are sets in Python?
Sets are unordered collections of unique elements used for efficient data handling.
Are sets in Python mutable?
Yes, sets are mutable, allowing modification by adding or removing elements.
What is the syntax of a set?
A set can be created using {}
or the set()
function.
Does set()
work for strings?
Yes, set()
can convert a string into a set of unique characters.
What is a frozenset in Python?
A frozenset
is an immutable version of a set, preventing modifications after creation.
What are set operations in Python?
Set operations include union, intersection, difference, and symmetric difference.
Conclusion
Mastering sets in Python is essential for efficient data handling. Their ability to store unique values, perform fast operations, and handle mathematical computations makes them invaluable in data science and programming. Whether you’re cleaning datasets, performing mathematical comparisons, or optimizing algorithms, sets provide a powerful solution.
Start Your Data Science Journey Today!
Learn more about Python and its applications in data science. Enroll in our Data Science Placement Program and kickstart your career with hands-on learning and guaranteed placement opportunities!
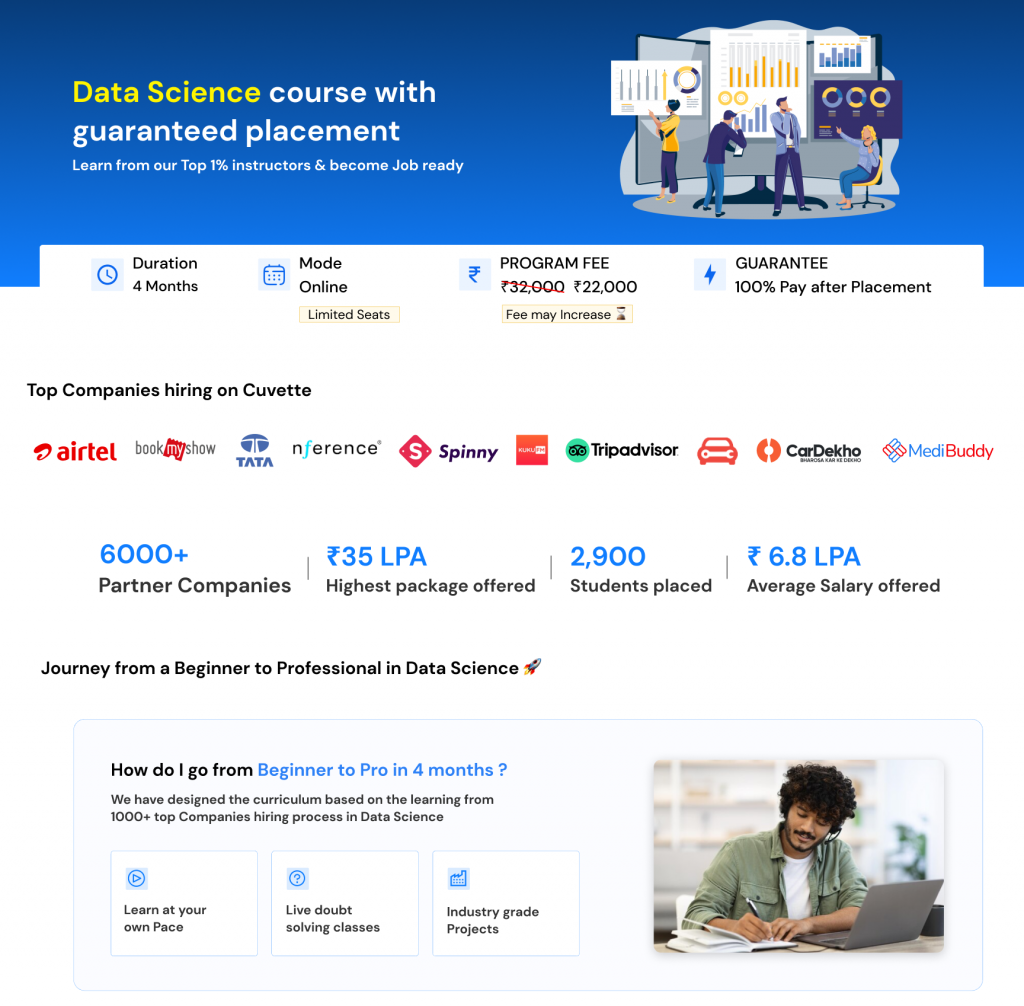
Recent Comments