Introduction
Java is a powerful object-oriented programming language widely used in software development. One of its key features is abstraction, which helps simplify complex systems by hiding implementation details. Abstract classes play a crucial role in achieving this. In this blog, we will explore abstract classes, their differences from interfaces, real-world applications, and best practices for their implementation.
What is an Abstract Class in Java?
An abstract class in Java is a class that cannot be instantiated on its own and must be extended by other classes. It is declared using the abstract
keyword and can contain both abstract (methods without a body) and non-abstract methods (methods with a body). Abstract classes help enforce a contract while allowing some level of implementation.
Key Features of Abstract Classes:
- Can have both abstract and concrete methods.
- Cannot be instantiated directly.
- Allows constructors and instance variables.
- Supports inheritance and method overriding.
Abstract Classes vs Interfaces in Java
Both abstract classes and interfaces provide abstraction but serve different purposes.
Feature | Abstract Class | Interface |
---|---|---|
Methods | Can have both abstract and concrete methods | Only abstract methods (until Java 8, after which default methods are allowed) |
Variables | Can have instance variables | Only static and final variables |
Constructors | Allowed | Not allowed |
Inheritance | Single inheritance | Multiple inheritance (through implementation) |
When to Use Abstract Classes Over Interfaces?
- When you need a base class with some default method implementations.
- When you want to use instance variables.
- When a strong hierarchical relationship exists among classes.
Real-World Usage of Abstract Classes
Abstract classes are widely used in Java applications. Some common scenarios include:
1. Frameworks and Libraries
Many Java frameworks, like Spring and Hibernate, use abstract classes to define base functionalities.
2. GUI Applications
Java’s Swing framework extensively uses abstract classes like AbstractAction
to define common UI behaviors.
3. Template Design Pattern
Abstract classes help implement the Template Method pattern, allowing subclasses to define specific steps of an algorithm while keeping the structure intact.
Industries Leveraging Abstract Classes
- E-commerce: Payment gateway integrations often use abstract classes to standardize transaction processes.
- Finance: Banking applications use abstract classes for transaction processing.
- Healthcare: Electronic medical record systems implement abstraction for different user roles.
- Gaming: Game engines use abstract classes to define behavior for different types of game objects.
Advantages of Abstract Classes Over Interfaces
- Code Reusability – Allows developers to reuse common functionalities across multiple subclasses.
- Flexibility – Supports partial implementations with concrete methods.
- Encapsulation – Provides a structured approach to defining class behavior.
- Performance – Abstract classes perform better than interfaces in certain scenarios due to single inheritance.
Implementing Multiple Inheritance with Abstract Classes
While Java does not support multiple inheritance with classes, it allows a combination of abstract classes and interfaces. By extending an abstract class and implementing multiple interfaces, developers can achieve the benefits of multiple inheritance.
Techniques and Limitations
- Using Interfaces: Implement multiple interfaces alongside an abstract class.
- Delegation: Use helper classes to manage functionality.
- Limitations: Java does not allow multiple class inheritance to prevent ambiguity.
Best Practices for Designing and Implementing Abstract Classes
- Keep abstraction meaningful: Avoid unnecessary abstraction.
- Use proper naming conventions: Names should indicate intent, e.g.,
AbstractShape
. - Provide necessary implementations: Use concrete methods where applicable.
- Use final keyword where needed: Protect certain methods from being overridden.
Structuring Abstract Classes
- Define common properties as instance variables.
- Use abstract methods for functionalities subclasses must implement.
- Implement utility methods in the base class.
Enhancing Code Reusability and Maintainability
Abstract classes contribute to cleaner and maintainable code by ensuring common functionalities are well-structured.
Benefits of Maintaining Code
- Reduces duplication.
- Improves readability and organization.
- Enhances scalability by allowing modifications in the base class.
Utilizing Static Methods in Abstract Classes
Abstract classes can have static methods, useful for utility functions that don’t depend on instance variables.
Practical Scenarios for Static Methods
- Utility methods for mathematical calculations.
- Logger methods for debugging.
Avoiding Common Pitfalls with Abstract Classes
- Avoid mixing unrelated functionalities in an abstract class.
- Do not force unnecessary abstraction.
- Ensure proper documentation and usage.
Key Mistakes to Avoid
- Creating abstract classes with no abstract methods.
- Overcomplicating inheritance hierarchies.
- Using abstract classes when interfaces would be a better choice.
FAQs
What is an abstract class in Java?
An abstract class is a class that cannot be instantiated and is meant to be extended by subclasses.
Why is it called an abstract class?
It is called ‘abstract’ because it defines a blueprint that subclasses must implement.
What is the benefit of using an abstract class?
It allows code reusability, better organization, and a structured way of defining common functionalities.
How to declare an abstract class in Java?
Use the abstract
keyword before the class definition, e.g., abstract class Vehicle {}
.
What is an abstract method in Java?
An abstract method is a method without a body that must be implemented by subclasses.
Can abstract classes be instantiated?
No, an abstract class cannot be instantiated directly.
What is the difference between an abstract class and an abstract method?
An abstract class is a class that may contain abstract methods, whereas an abstract method is a method without a body.
How are abstract classes used in practice?
They are used in frameworks, design patterns, and applications requiring code reusability.
Conclusion
Abstract classes are essential for structuring Java applications. They enable efficient code reuse, enforce design principles, and provide a foundation for scalable software development. Understanding when and how to use them effectively can significantly enhance your programming skills.
Want to master Java and other web technologies? Check out our MERN Stack course and kickstart your career!
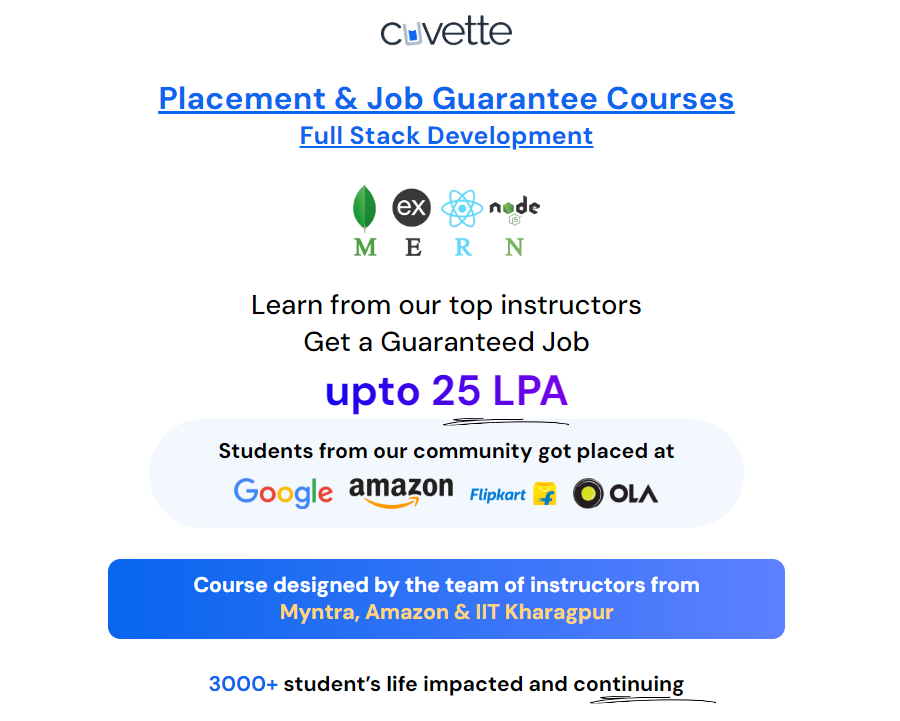
Recent Comments