Regular expressions, or regex, are one of the most powerful tools in JavaScript. They help developers match, search, and manipulate text efficiently. Whether you’re validating user input, searching for patterns, or transforming strings, regex is an essential skill. In this guide, you’ll learn everything from the basics of regex to advanced techniques.
If you are also looking for jobs or taking the first step in your web development career, join our Placement Guaranteed Course designed by top IITians and Senior developers & get a Job guarantee of CTC up to 25 LPA – https://cuvette.tech/placement-guarantee-program
Understanding the Basics of Regex in JavaScript
At its core, regex is a sequence of characters that defines a search pattern. In JavaScript, regular expressions are commonly used for input validation, parsing, and replacing text. They allow developers to match patterns efficiently, making string operations much simpler and faster.
Key Components of Regex:
- Literals: Exact characters to be matched.
- Metacharacters: Special symbols like
.
(any character),*
(zero or more repetitions), and+
(one or more repetitions). - Character Classes:
[a-z]
matches any lowercase letter,[0-9]
matches any digit. - Anchors:
^
(start of a string),$
(end of a string). - Quantifiers: Define how many times a character or group appears (e.g.,
{2,5}
means between 2 and 5 times).
Regex is widely used for input validation, such as verifying email addresses, phone numbers, and passwords. Mastering it will significantly improve your JavaScript skills.
Creating Regex Patterns
There are two primary ways to create a regex pattern in JavaScript:
- Using Regex Literals:
let pattern = /hello/;
- Using the RegExp Constructor:
let pattern = new RegExp("hello");
The regex literal is preferred for static patterns because it offers better performance. However, when working with dynamic patterns, the RegExp
constructor is more useful.
Using Regex Methods
Here’s a breakdown of some essential regex methods in JavaScript:
Method | Description | Example |
---|---|---|
test() | Checks if a pattern exists in a string (returns true or false ). | /hello/.test("hello world") |
exec() | Returns the first match found. | /\d+/.exec("Order 1234") |
match() | Returns all matches in a string. | "hello world".match(/o/g) |
replace() | Replaces matched patterns with new text. | "hello world".replace(/hello/, "Hi") |
These methods provide flexibility when working with text manipulation tasks.
If you are also looking for jobs or taking the first step in your web development career, join our Placement Guaranteed Course designed by top IITians and Senior developers & get a Job guarantee of CTC up to 25 LPA – https://cuvette.tech/placement-guarantee-program
Flags and Modifiers
Regex patterns can be modified using flags:
g
(global): Finds all matches instead of stopping at the first match.i
(case-insensitive): Makes pattern matching case-insensitive.m
(multiline): Allows^
and$
to match the start and end of each line.
Example:
let regex = /hello/gi;
let result = "Hello HELLO hello".match(regex); // ["Hello", "HELLO", "hello"]
Dynamic Regex Patterns
Dynamic regex patterns are useful when patterns need to change at runtime:
let userInput = "hello";
let regex = new RegExp(userInput, "i");
console.log(regex.test("Hello world")); // true
This is particularly helpful in form validation and search functionalities.
Common Regex Patterns
Pattern | Purpose | Example |
---|---|---|
/\d+/ | Match digits | "abc123".match(/\d+/) |
/[a-z]+/ | Match lowercase letters | "JavaScript".match(/[a-z]+/g) |
/^\w+@\w+\.\w+$/ | Validate email | "[email protected]".match(/^\w+@\w+\.\w+$/) |
Mastering these patterns will help you write more efficient code.
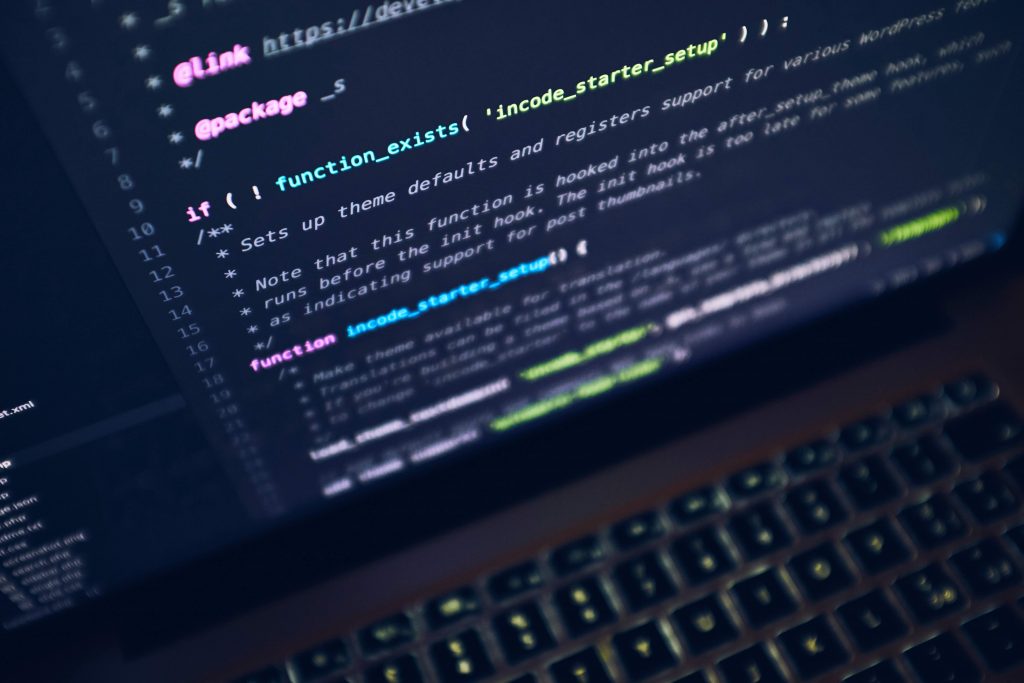
Debugging and Troubleshooting
Debugging regex can be tricky. Here are some tips:
- Use regex101.com to visualize patterns and test cases.
- Break complex patterns into smaller parts.
- Check for unnecessary escape characters.
If your regex isn’t working as expected, break it down into simpler pieces and test each separately.
Performance Optimization
Regex can slow down applications if not optimized. Here are some strategies:
- Use specific patterns instead of
.*
. - Avoid backtracking-heavy patterns.
- Precompile regex patterns when used frequently.
For example, instead of /.*hello.*/
, use /\bhello\b/
to match the word “hello” more efficiently.
Advanced Regex Techniques
Advanced techniques include:
- Capturing Groups: Extract parts of matched text (
/(\d+)-(\d+)/.exec("2024-02")
). - Lookaheads/Lookbehinds: Match without consuming characters (
/\d+(?= dollars)/
finds numbers before “dollars”). - Named Groups: Label parts of the match (
/(?<year>\d{4})-(?<month>\d{2})/.exec("2024-02")
).
Conclusion
Regex is a crucial tool for JavaScript developers. It enables pattern matching, text validation, and efficient string manipulations. Whether you’re building a form validation system, parsing text, or cleaning up data, mastering regex will boost your coding efficiency.
If you are also looking for jobs or taking the first step in your web development career, join our Placement Guaranteed Course designed by top IITians and Senior developers & get a Job guarantee of CTC up to 25 LPA – https://cuvette.tech/placement-guarantee-program
Start using regex today, and enhance your JavaScript skills with powerful pattern-matching techniques!
Recent Comments